A quick on using stb_truetype.h to render text in OpenGL using an alpha mask texture with the stbtt_PackBegin
/stbtt_PackFontRage
API.
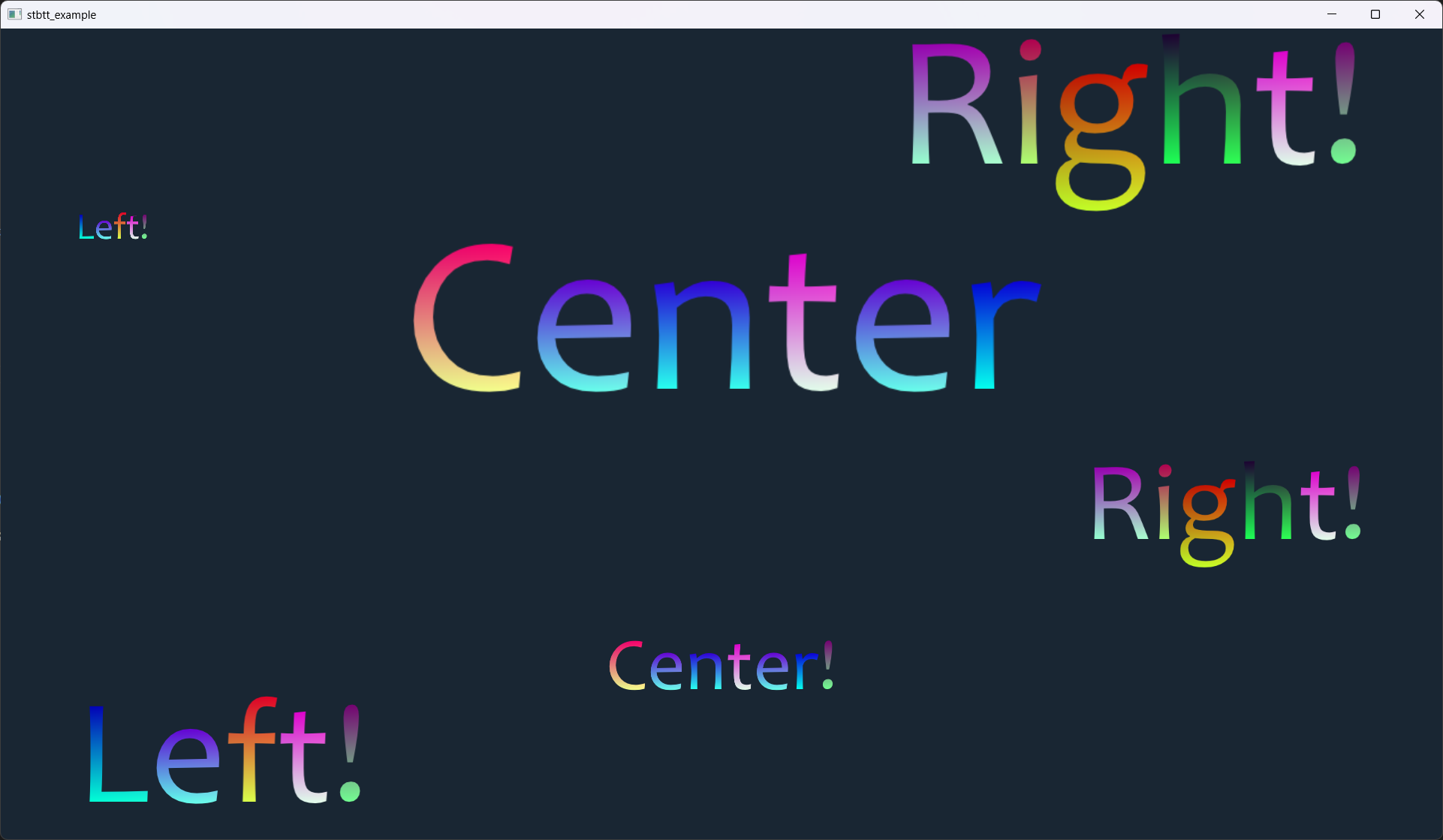
First load the font. The important data is the pixel data for the texture and packed character data. The example below packs characters from codepoints 0 to 125. If a different starting point than 0 is used then lookups in charData array must be offset by that number. (With 0-125 uppercase A will be at charData[65]
, with 32-125 then A is at charData[65-32]
.
stbtt_pack_context packContext;
stbtt_packedchar charData[126];
unsigned char pixels[TEXTURE_WIDTH * TEXTURE_HEIGHT];
stbtt_PackBegin(&packContext, pixels,
TEXTURE_WIDTH, TEXTURE_HEIGHT,
TEXTURE_WIDTH, 1, NULL);
stbtt_PackFontRange(&packContext, (unsigned char*)ttfData, 0, TEXTURE_FONT_SIZE,
0, 125, charData);
stbtt_PackEnd(&packContext);
Then put the pixel data into an OpenGL texture. The data is a single channel monochrome color:
glTexImage2D(GL_TEXTURE_2D, 0, GL_R8,
TEXTURE_WIDTH, TEXTURE_HEIGHT, 0,
GL_RED, GL_UNSIGNED_BYTE, pixels);
To render text, lookup each character in the charData array to get the position in the texture as char.x0, char.x1, char.y0, char.y1
. The actual size of the character can be calculated from the offsets as char.xoff2 - char.xoff
and char.yoff2 - char.yoff
. The xoff values are the start and end offset from the left. Yoff are the start and end offset from the baseline (y values will be negative when above the baseline, positive when below).
The x distance to the next character is available in char.xadvance
. This can also be used to calculating the full width of a string before rendering for things like scaling to fit or center/right aligning text.
MIT licensed demo code available here.